Picture this scenario: It's 2025 and you need real-time product data, daily web analytics, or robust market insights. Your challenge? Making sense of the boundless information scattered across the internet. That's where web scraping in R comes in. Through R's ecosystem, you can fetch, parse, and harness dynamic content in ways that would otherwise eat up your entire week.
Below, you will find a fully loaded guide to scraping the web with R in 2025. It is with a mix of code snippets, strategic tips, and best practices.
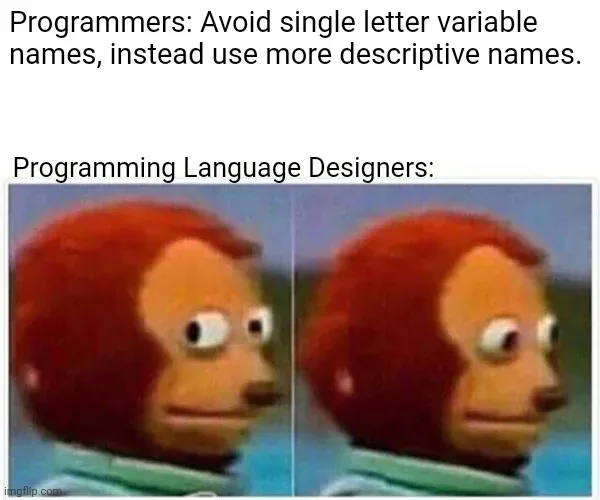
By the end, you will know which packages to install, how to handle hidden HTML elements, how to rotate user-agents, and even how to gracefully fail (or bypass minor blocks) using a touch of stealth.
Ready? Let's dive in.
1. Why R for Web Scraping?
You might be thinking, "Why is not Python the default web-scraping champion?"
If you are an R enthusiast, you already know R's data-wrangling talents are second to none. Combine that with rvest, httr, or RSelenium, and you get a scraping toolbox that stands comfortably alongside any mainstream Python solution.
- Data pipelines become simpler in R. Once you gather data with rvest, you can flow seamlessly into tidyr or dplyr for quick cleaning.
- Plotting results is a no-brainer, thanks to ggplot2.
- RMarkdown offers easy reporting.
No context-switching, no juggling multiple languages. Just load your libraries, spin up your script, and watch the magic happen.
2. Setting Up Your R Environment
Before typing a single line of code, let’s handle the environment. Make sure you have installed the necessary packages:
• base R (≥ 4.3.0 recommended)
• rvest (for HTML scraping)
• httr (for advanced HTTP requests)
• xml2 (underlying parsing)
• data.table or dplyr (fast data wrangling)
Your first step is to open up R or RStudio:
install.packages(c("rvest", "httr", "xml2", "data.table", "dplyr"))
You are good to go. Let's see some code.
3. Quick Win: Scraping Static Pages with rvest
If you want to scrape straightforward, static HTML pages, rvest has the goods.
Let's say we want to pull the headlines from a news site that still uses traditional HTML DOM without heavy JavaScript.
library(rvest)
library(dplyr)
url <- "https://example-news-website.org"
html_page <- read_html(url)
# Extracting headlines
headlines <- html_page %>%
html_elements(css = "h2.headline") %>%
html_text(trim = TRUE)
print(headlines)
Bang.
You get a neat vector of headlines ready for analysis. Notice we used the CSS selector h2.headline. Inspect the site, find the relevant tag or CSS class, and pass that to html_elements().
Why it works in 2025: Many sites remain partially static or have static subpages. If you pick the right DOM element, you will skip the hustle of dynamic rendering.
Handling JavaScript-Heavy Sites (and the RSelenium Detour)
But what about a site that loads content dynamically with JavaScript?
The typical read_html approach might yield empty nodes.
Enter RSelenium.
• RSelenium launches a real browser instance (such as Chromium) within R.
• You can navigate to the page, let the JavaScript load, then scrape the final rendered DOM.
Example snippet:
install.packages("RSelenium")
library(RSelenium)
# Start a local Selenium server (Chromedriver, in this example)
selService <- rsDriver(browser="chrome", port=4545L)
remoteDriver <- selService$client
remoteDriver$navigate("https://dynamic-website.org")
# Wait a moment for JS to load
Sys.sleep(3)
# Retrieve page source
page_source <- remoteDriver$getPageSource()[[1]]
doc <- read_html(page_source)
# Extract elements
items <- doc %>%
html_elements(".item-title") %>%
html_text()
print(items)
The Sys.sleep(3) ensures you give the site time to render the dynamic content.
Of course, you can replace that static wait with more robust solutions, like a quick check for known elements. Once done, RSelenium fetches a final, fully-loaded DOM.
Managing Rate Limits and User-Agents
Many sites have grown suspicious in 2025. They see repeated requests from the same user-agent or IP, and they clamp down. The best approach? Rotate user-agents, or throttle your requests to appear more human.
Below is a quick approach using the httr package. We rotate through an array of user-agents:
ibrary(httr)
user_agents <- c(
"Mozilla/5.0 (Windows NT 10.0; Win64; x64)",
"Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7)",
"Mozilla/5.0 (Linux; Android 11)"
)
for(i in seq_along(user_agents)) {
custom_header <- add_headers("User-Agent" = user_agents[i])
Sys.sleep(2) # be polite
res <- GET("https://some-website.org/products", custom_header)
html_doc <- read_html(content(res, "text"))
# parse data ...
}
You can combine that with a random wait time (runif(1, 1.5, 3.0)) to evade basic detection. If you require IP rotation, look into proxies. Instead of simply connecting to a single IP, you can pass a different proxy on each request to appear like multiple distinct visitors. Proxies can be set via arguments in GET() or read_html().
Cookies and Session Handling
When sites rely on login sessions, you must store cookies. The httr package has built-in cookie handling. You can create a session with session <- session_info(), log in once, and maintain that session cookie for subsequent requests.
For example:
session <- html_session("https://login-required-site.org/login")
form <- html_form(session)[[1]]
filled_form <- set_values(form,
"username" = "myUserName",
"password" = "mySuperSecretPassword"
)
logged_in <- submit_form(session, filled_form)
# session now holds your cookies
content_page <- jump_to(logged_in, "https://login-required-site.org/secret/data")
parsed <- read_html(content_page) %>%
html_elements(".secret-data") %>%
html_text()
Yes, web scraping in 2025 remains extremely powerful, but please be ethical:
- Always check robots.txt for scraping allowances.
- Add delays between requests.
- Don't overload servers.
Respect sites that block or reject your requests.
Remember, scraping a site against its TOS can lead to IP bans or legal trouble.
Tread carefully, keep each site's guidelines in mind, and try to keep your request volume minimal.
Bypassing Basic Blocks or Obfuscation
Occasionally, sites will embed minor obfuscation in their HTML or rely on dynamic links to hamper scraping.
A quick fix might be to run JavaScript in a headless environment (like RSelenium or a Docker-based Puppeteer container).
However, a short-coded approach is to parse the inline JavaScript:
library(stringr)
script_nodes <- html_doc %>%
html_elements("script") %>%
html_text()
# Suppose there's a snippet: var realURL = "hxxp://mysite.org"+"/" + "hidden123"
for(scr in script_nodes) {
result <- str_match(scr, "var realURL\\s*=\\s*\"(.*?)\"\\+\"(.*?)\"\\+\"(.*?)\"")
if(!is.na(result[1])){
link <- paste0(result[2], result[3], result[4])
print(paste("Extracted Link:", link))
}
}
The snippet above uses a regular expression to parse out a concatenated string.
Hacky, sure, but it can salvage data that tries to hide behind trivial JavaScript manipulations.
Sneaky Approaches (If You Must)
Sometimes, you need an extra edge to get data from sites that do IP-based blocks or partial Cloudflare checks.
Tools like cloudscraper exist in Python, but R can leverage packages like V8 or system calls to run them as well. You can script a tiny Python snippet from R:
library(reticulate)
py_run_string("
import cloudscraper
scraper = cloudscraper.create_scraper()
resp = scraper.get('https://some-cloudflare-protected-site.org')
print(resp.text)
")
While not a universal solution, the above approach can help you gather HTML that standard requests block.
Post-Processing: Data Storage and Visualization
Once you collect your data, you need to store it or visualize it. Because you’re already in R, the pipeline is straightforward:
• Convert your data into a data.frame (or tibble).
• Save it to CSV or a local database.
• Cleanup with dplyr.
• Plot with ggplot2 if needed.
Here is a quick snippet:
df <- data.frame(
product = items,
price = prices,
rating = ratings
)
write.csv(df, "scraped_data.csv", row.names=FALSE)
# Analyze
df %>%
filter(price < 20) %>%
arrange(desc(rating))
Time to highlight those bargains you just discovered.
Wrapping Up
Web scraping in R has evolved big-time by 2025. It thrives on powerful packages (rvest, httr, RSelenium) and nutrients from the R ecosystem (tidyverse, data.table, reticulate).
You can rotate user-agents, parse JavaScript, manage cookies, and even slip around simplistic blocks. And once your data is locked down, you can spin it into graphs, dashboards, or machine-learning pipelines.
It's a reminder that R, once labeled "The quirky stats language" stands tall beside Python or JavaScript for real-world data tasks.
If used ethically, scraping can give you essential insights while saving you ridiculous amounts of time. And as the web matures and transforms, you will have the perfect stash of R-based approaches in your back pocket.
So pass around that snippet to your co-workers. Or keep it hush-hush. Because in the data realm of 2025, a little scraping know-how can open big doors.