From flexible setup to lightning-fast performance, Playwright has become a go-to solution for automated browser testing.
This post will show you how to install, structure, and run your first tests with Playwright, using short, simple steps.
You’ll also see how to customize your workflow to suit real-world scenarios.
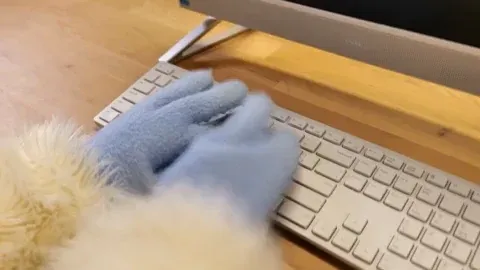
In each section, we’ll do a post-mortem of best practices, share some example code, and round off with a couple of tips on making the most of the library.
Sound good? Let’s jump in.
What is Playwright, and Why Should You Use It?
Playwright is an open-source testing library developed by Microsoft.
It’s designed to automate modern web applications across multiple browsers—including Chromium, Firefox, and WebKit.
You can test for functionality, responsiveness, and performance, all in one place.
Its cross-browser coverage sets it apart from many other test frameworks.
One test suite can run on a wide array of environments, so your QA (Quality Assurance) pipeline simplifies substantially.
It excels in:
• Speed and reliability.
• Full browser coverage.
• Handy features like auto-waiting and screenshot captures.
If you’ve struggled with flaky tests in the past, Playwright’s architecture may well be the remedy you need.
Setting Up Your Playwright Environment
Playwright is built for Node.js, though you can use languages like Python or .NET if you prefer.
Here, we’ll stick to Node.js.
First, initialize your project:
mkdir playwright-demo
cd playwright-demo
npm init -y
This sets up a basic package.json.
Next, install Playwright:
npm install -D @playwright/test
The “-D” flag ensures the package is stored in devDependencies, which is a best practice for test libraries.
After that, you can install the browsers:
npx playwright install
This single command grabs the latest versions of Chromium, Firefox, and WebKit.
Writing Your First Playwright Test
You can store tests in a “tests” folder, or wherever you prefer, as long as you configure it in the playwright.config file later.
Create a file named “example.spec.js” inside a folder called “tests”:
// tests/example.spec.js
const { test, expect } = require('@playwright/test');
test('Basic navigation test', async ({ page }) => {
// Go to a site
await page.goto('https://example.com');
// Check page title
await expect(page).toHaveTitle(/Example Domain/);
// Interact with page
const moreInfoLink = page.locator('a:has-text("More information")');
await moreInfoLink.click();
// Assert new URL
await expect(page).toHaveURL(/iana.org/);
});
Let’s break it down:
• The test() function defines a single test. You pass a callback that receives the page object.
• page.goto() navigates to the target site.
• expect(page).toHaveTitle() checks the page title matches a pattern or exact string.
• page.locator() finds a clickable element with certain text.
• moreInfoLink.click() triggers the user action.
• expect(page).toHaveURL() verifies the new URL.
These short commands illustrate how intuitive Playwright’s syntax is.
Configuring Your Test Runner
By default, @playwright/test includes a built-in test runner.
You can customize it in the “playwright.config.js” file at the root of your project:
// playwright.config.js
module.exports = {
testDir: './tests', // location of test files
timeout: 30000, // 30-second test timeout
retries: 2, // retry failing tests up to 2 times
use: {
headless: true, // run tests in headless mode
screenshot: 'only-on-failure',
video: 'retain-on-failure',
},
};
Some noteworthy config keys:
• testDir points to your test files.
• timeout sets the maximum time each test can run before failing.
• retries automatically reruns failing tests for resilience.
• headless toggles the browser UI.
• screenshot and video settings capture artifacts for debugging.
Running Your Tests
Once you have your spec file and config in place, run:
npx playwright test
Playwright will detect the tests in your “tests” folder, spin up the browsers, and display results in the terminal.
For each test, you’ll see a pass/fail status, along with a summary of how many total tests succeeded.
If you want to run only a subset of tests, pass the file name:
npx playwright test tests/example.spec.js
Or run with debug:
npx playwright test --debug
Debug mode opens the inspector, letting you watch the browser as the steps unfold.
This mode also offers step-by-step trace viewing, so you can see exactly where an error might occur.
Parallelizing Tests
Playwright supports parallel execution out of the box.
In large projects, you’ll often have dozens of tests, so it’s beneficial to distribute them across multiple worker processes.
Adjust concurrency in your config or via CLI:
npx playwright test --workers=4
With four workers, the test runner schedules tasks in parallel.
Your test suite can complete faster, although you may need to manage shared state carefully to avoid collisions.
Using Fixtures and Hooks
If you need to log in users, set up data, or perform any repeated action for multiple tests, you can harness fixtures or hooks.
For instance, you can define a “beforeAll” step that populates your database:
test.beforeAll(async () => {
// Insert mock user
await mockUserDatabase.insert({ name: 'Alice', status: 'active' });
});
Then, in each test, that user is guaranteed to exist.
Likewise, test.afterAll can tear down data once you’re finished.
Handling Screenshots and Traces
One of Playwright’s biggest perks is its debugging capabilities.
You can record screenshots by default whenever a test fails.
To enable them, you already saw the “screenshot: ‘only-on-failure’” line in your config.
For advanced debugging, toggle the trace option:
use: {
trace: 'on-first-retry'
}
If a test fails on the first try, the second run captures a detailed trace.
This trace can then be opened in the Playwright Trace Viewer, showing every step, console log, network request, screenshot, and more.
Quick Learnings
- Keep tests isolated: Let each spec manage its own data or environment to reduce flakiness.
- Standardize your config: A stable configuration ensures consistent runs.
- Use test fixtures for repeated tasks: This DRYs up your code.
- Debugging is your friend: Lean on the inspector, trace viewer, and screenshots.
- Build gradually: Start with simpler end-to-end checks, then ramp up to scenario-based tests.
Scaling Your Playwright Workflow
As your project grows, keep a few tips in mind:
• Use environment variables: For credentials or environment toggles.
• Integrate with CI: Tools like GitHub Actions or Jenkins let you automate and schedule test runs.
• Report results: Store screenshots, videos, and logs for quick reference.
• Tag your tests: Mark some tests as “smoke” or “regression” to manage suite size in CI.
In corporate settings, you can also connect Playwright to containerized infrastructure.
Spinning up ephemeral Docker containers for each test cycle keeps your runs pristine.
Final Thoughts
Playwright stands out for its multi-browser coverage, debugging power, and straightforward syntax.
It transforms how developers create and maintain end-to-end tests.
You can navigate the complexities of modern web apps with minimal friction, all while capturing logs, screenshots, and videos for clarity.
Whether you’re working on a small side project or a large enterprise platform, Playwright’s reliability will help speed up QA cycles and catch regressions early.
Give it a try, and see if it brings that extra bit of confidence to your release pipeline.
Remember, the best test frameworks aren’t just about functionality—they’re about creating a seamless development experience.
With Playwright, you get both coverage and convenience, wrapped in a friendly API.
Hopefully, this gives you the foundations you need.
Now it’s your turn to put it into practice and refine your end-to-end tests.
Happy testing :)